일 | 월 | 화 | 수 | 목 | 금 | 토 |
---|---|---|---|---|---|---|
1 | 2 | 3 | 4 | 5 | ||
6 | 7 | 8 | 9 | 10 | 11 | 12 |
13 | 14 | 15 | 16 | 17 | 18 | 19 |
20 | 21 | 22 | 23 | 24 | 25 | 26 |
27 | 28 | 29 | 30 |
- 이진 변환 반복하기
- 셀레니움
- 파이썬
- 메모장
- android
- n^2 배열 자르기
- 프로그래밍
- jsp
- jdbc
- 유튜브
- 크롤링
- Programmers
- 세션
- 코딩
- js
- 함수형 인터페이스
- 파일 저장
- 입출력
- 모바일
- java
- MySQL
- 프로그래머스
- javascript
- 개발자
- Python
- 데이터베이스
- 형태소 분석기
- 자바스크립트
- 개발
- 자바
- Today
- Total
개인용 복습공간
[JSP] JDBC로 데이터베이스 연동 - 1 (스크립트릿) 본문
프로그램에서 데이터베이스를 연동하기 위한
자바의 기술인 JDBC에 대해서 알아본다.
스크립트릿으로 데이터베이스 연동하는 법을 알아본다.
JDBC
JBDC (Java DataBase Connectivity)란?
데이터 베이스를 다루기 위한 자바 API (Application Programming Interface)이다.
JDBC의 구성
- JDBC 인터페이스 : 프로그래머에게 쉬운 데이터베이스와 연동되는 프로그램을 작성할 수 있게 하는 도구
- JDBC 드라이버 : JDBC 인터페이스를 구현하여 실제로 DBMS를 작동시켜서 질의를 던지고 결과를 받음
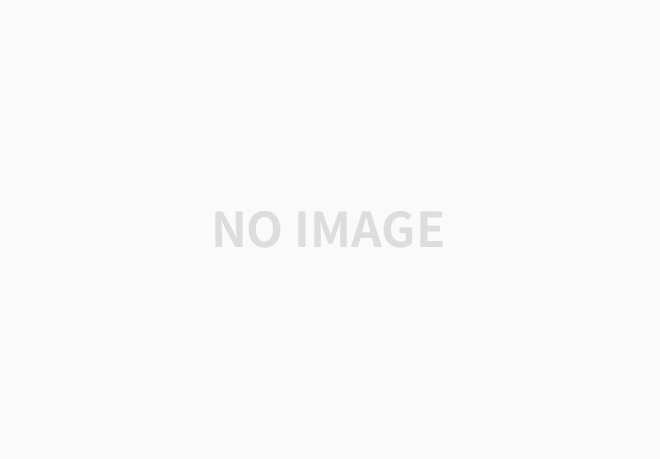
JDBC Driver 설치
MySQL 사이트 (http://dev.mysql.com/downloads/connector/j/ )
mysql-connector-java-8.0.20.zip 다운로드 후, 압축해제 (최신 버전 8.0.24)
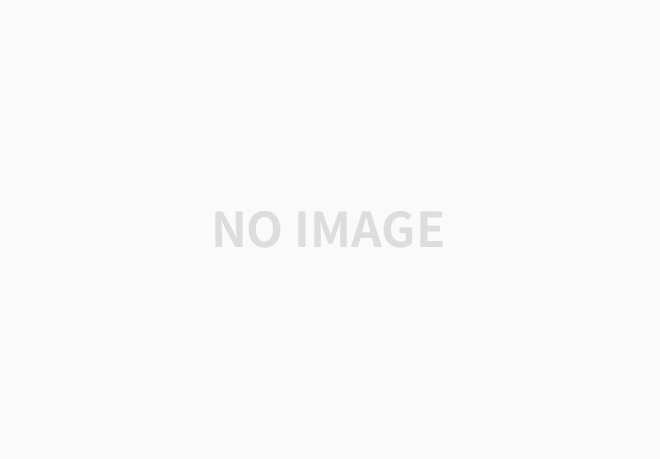
mysql-connector-java-8.0.20.jar를 다음 폴더 중 하나에 복사한다.
- [Tomcat 설치 폴더]/lib
- program files/Java/JreX/lib/ext
- 이클립스 라이브러리 추가
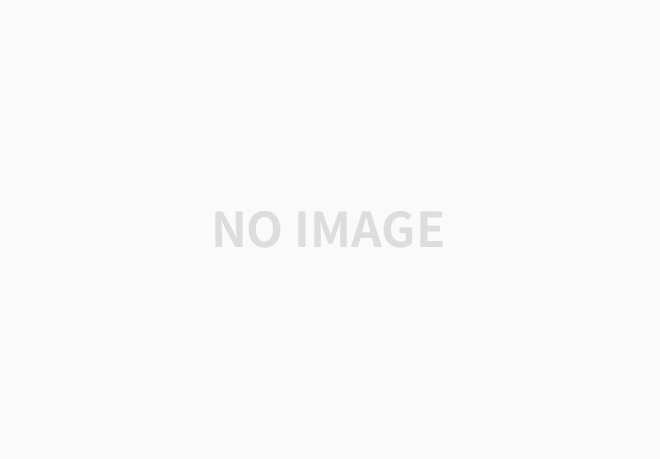
이클립스에서 JDBC 드라이버 설치 확인
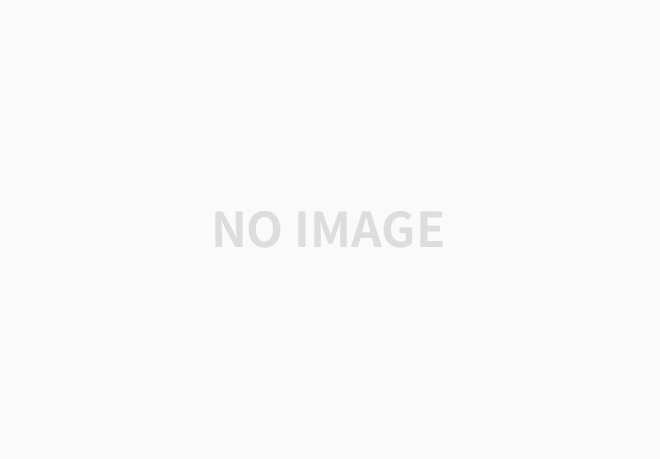
JDBC를 통한 MySQL과의 연동 테스트
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
|
import java.sql.*;
public class DriverTest{
public static void main(String args[]){
Connection con = null;
try{
Class.forName("com.mysql.cj.jdbc.Driver");
con=DriverManager.getConnection(
"jdbc:mysql://localhost:3306/mydb?serverTimezone=UTC",
"root", "1234");
System.out.println("Success");
}
catch(SQLException ex){
System.out.println("SQLException" + ex);
ex.printStackTrace();
}
catch(Exception ex){
System.out.println("Exception:" + ex);
ex.printStackTrace();
}
}
}
|
cs |
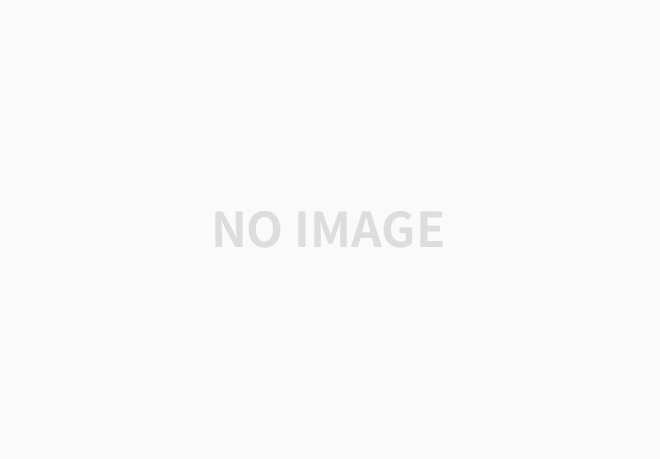
데이터베이스 조작을 위한 자바 라이브러리
JDBC API (java.sql 패키지)
- Driver : 모든 드라이버 클래스들이 구현해야 하는 인터페이스
- DriverManager : 드라이버를 로드하고 데이터베이스에 연결
- Connection : 특정 데이터베이스와의 연결
- Statement : SQL문을 실행해 작성된 결과를 반환
- PreparedStatement : 사전에 컴파일된 SQL문을 실행
- ResultSet : SQL 문에 대한 결과를 얻어냄
JDBC 프로그래밍 단계
1. JDBC 드라이버의 인스턴스 생성
1
|
Class.forName("Driver_Name");
|
cs |
드라이버 이름은 MySQL 8.0 경우 com.mysql.cj.jdbc.Driver
2. JDBC 드라이버 인스턴스를 통해 DBMS에 대한 연결 생성
1
|
Connection con = DriverManager.getConnection("DBURL", "Account ID", "Account PW");
|
cs |
getConncetion에는 연결 DBURL과 데이터베이스의 user id, password를 작성해준다.
3. Statement 생성
1
|
Statement stmt = con.createStatement();
|
cs |
Statement는 단순한 sql 문장 보낼 때 사용한다. 성능과 효율성이 낮다.
PreparedStatement는 여러 번 반복해서 사용되는 SQL을 다룰 때 편리하다.
ex) PreparedStatement pstmt = con.prepareStatement(SQL);
4. 질의문 실행/ResultSet으로 결과 받음 (executeQuery, executeUpdate)
1
|
ResultSet rs = stmt.executeQuery("select * from ...");
|
cs |
ResultSet는 테이블 형태의 결과를 추상화한 인터페이스이다.
executeQuery는 select 문을 쓸 수 있고 executeUpdate는 create, drop, insert, delete, update 문을 사용할 수 있다.
5. ResultSet 해지 (자원 해지)
1
|
rs.close();
|
cs |
6. Statement 해지
1
|
stmt.close();
|
cs |
7. 데이터베이스와 연결 해지
1
|
con.close();
|
cs |
스크립트릿으로 데이터베이스 연동 예제
JSP 스크립트릿으로 데이터 베이스를 연동하여 mydb.tblregister 테이블에 있는 레코드를 조회한다.
usingJDBCjsp.jsp
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
43
44
45
46
47
48
49
50
51
52
53
54
55
56
57
58
59
60
61
62
63
64
65
66
67
68
69
70
71
72
73
74
75
76
77
78
79
80
81
82
83
84
85
86
87
88
89
90
91
92
93
94
95
96
97
98
99
100
101
102
103
104
105
106
107
108
109
110
111
|
<%@ page language="java" contentType="text/html; charset=EUC-KR"
pageEncoding="EUC-KR"%>
<%@ page import="java.sql.*" %>
<%
Class.forName("com.mysql.cj.jdbc.Driver");
Connection conn = null;
Statement stmt = null;
ResultSet rs = null;
String id="";
String pwd="";
String name="";
String num1="";
String num2="";
String email="";
String phone="";
String zipcode="";
String address="";
String job="";
int counter = 0;
try{
conn=DriverManager.getConnection(
"jdbc:mysql://localhost:3306/mydb?serverTimezone=UTC",
"root", "1234");
stmt = conn.createStatement();
rs = stmt.executeQuery("select * from tblregister");
%>
<!DOCTYPE html>
<html>
<head>
<meta charset="EUC-KR">
<title>JSP에서 데이터베이스 연동</title>
<link href="style.css" rel="stylesheet" type="text/css">
</head>
<body>
<div align="center">
<h2>JSP 스크립트릿에서 데이터베이스 연동 예제</h2>
<h3>회원정보</h3>
<table>
<tr>
<th>ID</th>
<th>PASSWD</th>
<th>NAME</th>
<th>NUM1</th>
<th>NUM2</th>
<th>EMAIL</th>
<th>PHONE</th>
<th>ZIPCODE/ADDRESS</th>
<th>JOB</th>
</tr>
<%
if(rs!=null){
while(rs.next()){
id = rs.getString("id");
pwd = rs.getString("pwd");
name = rs.getString("name");
num1 = rs.getString("num1");
num2 = rs.getString("num2");
email = rs.getString("email");
phone = rs.getString("phone");
zipcode = rs.getString("zipcode");
address = rs.getString("address");
job = rs.getString("job");
%>
<tr>
<th><%=id %></th>
<th><%=pwd %></th>
<th><%=name %></th>
<th><%=num1 %></th>
<th><%=num2 %></th>
<th><%=email %></th>
<th><%=phone %></th>
<th><%=zipcode %> / <%=address %></th>
<th><%=job %></th>
</tr>
<%
counter++;
}//end while
}//end if
%>
</table>
total records: <%=counter %>
</div>
<%
}catch(Exception ex){
ex.printStackTrace();
}finally{
if(rs!=null){
try{
rs.close();
}catch(Exception e){}
}
if(stmt!=null){
try{
stmt.close();
}catch(Exception e){}
}
if(conn!=null){
try{
conn.close();
}catch(Exception e){}
}
}
%>
</body>
</html>
|
cs |
이와 같이 JSP 페이지 내에서 직접 데이터베이스 관련 코드가 혼재되어 있어 추후 페이지 변경 등의 작업이 있을 때 유리하지 못하다.
스크립트릿으로 데이터베이스 연동 예제 실행화면
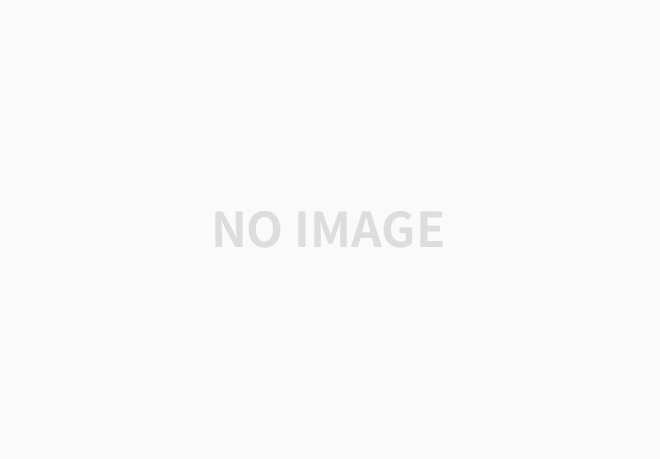
다음은 자바 빈즈를 이용한 데이터베이스 연동을 해볼 것이다
'웹 > Jsp, Servlet' 카테고리의 다른 글
[Jsp] 세션과 쿠키 - (2) (0) | 2021.06.01 |
---|---|
[Jsp] 세션과 쿠키 - (1) (0) | 2021.05.28 |
[Jsp] JDBC로 데이터베이스 연동 - 3 (회원관리 만들기) (0) | 2021.05.27 |
[JSP] JDBC로 데이터베이스 연동 - 2 (자바빈즈, Connection Pool) (0) | 2021.05.13 |